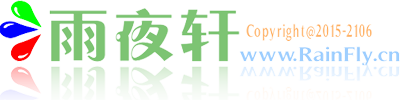
公告:
1. 下载 PL/SQL 工具
2.解压并安装对应工具
3. 注册激活工具 (利用附件中的汉化工具)
4.新增链接实例
5. 设置编辑器显示字体,为了看起来舒服点。
7. 相关工具使用
...阅读全文>>
之前一直使用mysql作为存储数据库,虽然中间偶尔使用sqlite作为本地数据库存储,hive作为简单查询工具,maxcompute作为大数据查询服务等等,但没有感觉多少差别。事实上,我们往往听说SQL-92标准之类的云云!
后来遇上了oracle,且以其作为主要存储,这下就不得不好好了解其东西了。oracle作为商业数据库里的佼佼者,肯...阅读全文>>
1。打开网盘下载以下资料:按照【Oracle 经典教程】进行安装 Oarcel 文档
2. 安装完成后打开配置工具进行配置。
配置连接出错,
Oracle错误:ORA-12541 TNS无监听程序 - MySQL
要注...阅读全文>>
简介:Navicat Premium是一套数据库开发工具,让你从单一应用程序中同时连接 MySQL、MariaDB、MongoDB、SQL Server、Oracle、PostgreSQL 和 SQLite 数据库。Navicat Premium与 Amazon RDS、Amazon Aurora、Amazon Redshift、Microsoft Azure、Oracle Cloud、MongoDB Atlas、阿里云、腾讯云和华为云等云数据库兼容。你可以快速轻松地创建、管理和维护数据库。
阅读全文>>
阿里巴巴Java开发手册是由阿里巴巴集团编写的一份规范文档,旨在提供一套统一的编码规范和最佳实践,以帮助Java开发人员提高代码质量和开发效率。
阿里巴巴Java开发手册嵩山版包含了丰富的内容,涵盖了Java语言的各个方面,例如命名规范、代码格式、注释规范、异常处理、并发编程、数据库访问等...阅读全文>>
联想拯救者R720安装黑苹果
CPU
核心显卡 Intel HD Graphics 630 2048 MB
独立显卡1050TI
蓝牙
声卡(支持HDMI音频输出)
WiFi
HDMI
睡眠唤醒 (...阅读全文>>
搜索一番,是由于IDEA版本导致的Lombok失效,不过这个问题后来解决了。
所以,我们只需要更新lombok版本,使用1.18.14及之后的版本即可:
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId...阅读全文>>
一. 创业初期
在创业初期,我是那个亲手做东西的人,而且全面负责,从理论到实现,从加工到调试,什么要做做什么,什么不会学什么。第一代原型机就是在我手上诞生的。那时候我非常兴奋,每天睡4-6个小时,不用闹钟,是兴奋醒的。
我既是技术副总,又是技术总监,又是部门主管,又是...阅读全文>>
1.首先先准备好 jdk1.7 linux 和tomcat7的安装包(这里使用的是jdk linux 32位的 文章底部奉上需要的安装包)
2. 将包文件拷贝到用户目录 我这里是 /home/rain/
3.解压 jdk文件 和tomcat文件
...阅读全文>>
外面的 html 文件 index.html:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>fullScreen</title>
...阅读全文>>
$("#roomNo").change(function() {
if ($("#roomNo").get(0).checked) {
alert("选中");
}else {
alert("取消");
$("#roomNo_span").remove();
...阅读全文>>